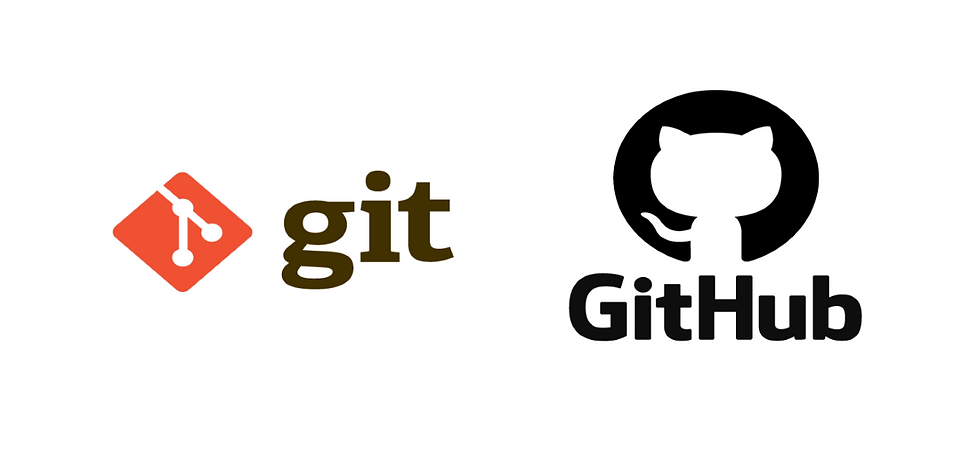
If you are interested in learning about Computer Science and Programming, I am sure you would have listened about this terms frequently. But If you have not yet started using it then this post will definately help you out. I myself was unaware about it. Then with some industrial Guidance and with the help of Things over Internet i found things more easier to get started with and I am presenting my knowledge to you. Let's begin about our Git and GitHub.
What is Git ?
Git is a VCS — Version Control System. What that really means is, Git helps us manage our project files. One of the primary things that git does and also the primary reason it exists is to keep track of the entire history of things that you are working on.
This is especially helpful for software developers because when you are working on a project you first build a basic version of it and then try to improve it by adding new features or just experiment with things. This whole process of experimenting with new features is incredibly error prone and you might wanna revert back to your original code.
This is where Version Control comes into play, it automatically tracks every minute change in your project and allows us to revert back to a previous version no matter how many times you changed your files.
Another awesome thing that Git allows to do is, it allows people to work together on the same project at the same time without disturbing each other’s files. Collaboration is all the more easier with Git.Team members can work on different features and easily merge changes.
Git is easy to learn and has a lightning fast performance. It outclasses other Version Control Systems like SubVersion with features like cheap and local branching, convenient staging areas and multiple workflows.
What is GitHub ?
GitHub is a web-based service for version control using Git. Basically, it is a social networking site for developers. You can look at other people’s code, identify issues with their code and even propose changes. This also helps you in improving your code. On a lighter note, it is a great place to show off your projects and get noticed by potential recruiters.
In short, Git is Version Control System and GitHub is a hosting service for Git Repositories.
Basic Terminologies
Repository: A Git Repository, or a repo, is a folder that you’ve told Git to help you track file changes.
Branch: A branch is an independent line of development. You can think of it as a brand new working directory.
Fork: A fork is a personal copy of another user’s repository that lives on your account.
Clone: A clone is simply a copy of a repository that lives on your computer instead of on a server.
Commit: A commit is a set of one or more changes to a file(or a set of files). Every time you save, it creates a unique ID(“hash”) which helps it keep track of the history.
Master: The default development branch. Whenever you create a git repo, a branch named “master” is created which becomes the default active branch.
Download Git on your System as per Specifications to continue further. (You can refer git-scm.com/downloads)
Now we will be starting with Git commands in Git Command Terminal.
Initial Set Up :
Since Git is designed to record changes to a global repository, it needs to be able to identify you and provide a means of contact. These steps are global, in that they will apply for all repositories you set up on your current local machine.
This information will be visible in all repositories you make public so don't use details you'd rather keep private.
Firstly, Right Click on the empty folder where you wish to save your git projects. After right click there will be option available for "Git Bash here ".
Click on it and it will open Git Command Terminal.
At your git terminal type:
git config --global user.name "Your name"
git config --global user.email "your.email@yourmailserver.com"
The command:
git config --list
will show you the existing Git config settings.
Task 1 : Creating a Repository
You're going to create a new folder and initialize it as a Git repository. Firstly, Right Click on the empty folder where you wish to save your git projects. After right click there will be option available for "Git Bash here ".
Click on it and it will open Git Command Terminal.
There is always an $ sign on every new line.
For creating the Git repository type in the code below
1.) To make a New Folder
$ mkdir <Repository Name>
For Eg : $mkdir NACTORE_Repo
2.) To go into the folder (Not Manually but with commands - more good practice )
$ cd <Repository Name>
For Eg : $ cd NACTORE_Repo
3.) To Create a new Git Instance for a project(make sure you performed 2nd step or else you may lose your file)
$ git init
This will now convert your Folder into a Git repository. To cross check your task verify it using git status verification code. If it returns 'fatal: Not a git repository...', It means that you made a mistake. Or elese you have successfully created the Repository.
$ git status
Task 2 : Create a new File and Commiting it.
Now on the created repository make any text file with suitable text editor. Write couple of lines of text and save the file with your desired filename Eg : NACTORE.txt
Make sure you create this file in the git repository created previously.
Before Implementing Codes in Git Command Line let up attempt to Understand the Working of File Inclusion within the Git Repository.
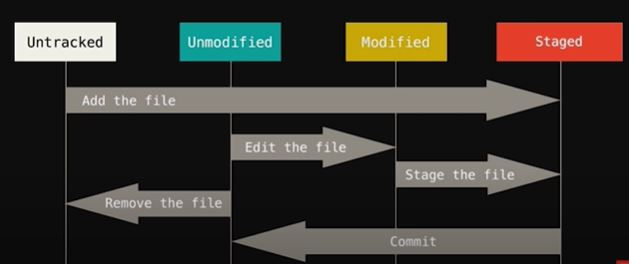
Files in a repository go through three stages before being under version control with git:
Untracked: the file exists, but is not part of git's version control
Staged: the file has been added to git's version control but changes have not been committed
Committed: the change has been committed
Git-status is used to understand what stage the files in a repository are at.
$ git status
The output of this command not only tells you the stage of the files in your repository but also gives you some handy tips on what to do next.
So our next stage is to add our files to the staging area:
$ git add NACTORE.txt
If we re-run the git status command at this point the output gets changed.
The final stage is to commit the change we have made. We do this by recording a short message that explains what we did and why. This human-readable explanation will go alongside Git's own record of the change and the file structure snapshot at that point.
$ git commit
This pops open your default text editor and asks for a commit message. Provide the Idea of what change we made to the file and then quit it. Now again on running git status code we must get No files to be commited , Working Tree Clean.
We can shorten these steps slightly using the -m flag to the git commit statement. Staging and committing can therefore be done in one go using the following syntax:
$ git commit -m"Changes you made in form of a comment "
Also, running git log will give you a nicely formatted record of your changes:
$ git log
Let's make a change to the file so we can try out some other commands...
In your text editor of choice, add a line to NACTORE.txt that says what your name is, and save the file.
We can view the difference between that version of the file and the committed one (eg the changes) using the following command:
$ git diff
Again we need to add this file to the staging area and then commit it's changes. Since the file is already in the repository, we can use another shortcut to add it and commit it in one go
$ git commit -am"Added name to NACTORE.txt"
Running git diff again should show no changes, as the file and repository are up to date.
One useful command in this section is the "--amend" flag for git commit, which allows you to amend the previous commit, for example to fix a spelling mistake.
$ git commit --amend
Ignoring Files
There are many scenarios where you might end up with files in your repository that you don't want to place under source control. These might be backup files that text editors create, compiled files or even files with sensitive information in, such as passwords. You can place these in a file called .gitignore (which will be hidden by default).
Removing files
Git uses the unix mv (move or rename) and rm (remove) commands, but also deals with the tasks of staging and unstaging the files too.
We use different rm commands depending on what we want to do.
$ rm filename : removes a file that hasn't been added to the repository yet- this will delete the file from your file system
$ git rm filename : removes the file from the repository and deletes it from your file system
$ git rm --cached filename : removes the file from the repository but doesn't delete it from your file system
Make Sure You are able to see the Repositories created on your System linked on your Git Hub Account. We shall Continue further by next Week. In case of any difficulties, Comment below in Thread itself. ✌✌
|| We are back again continuing the Thread with GitHub Initialization Process ||
Remote and Push
Online versions of repositories are generally known as remotes, and the process of uploading code to a remote is known as a push.
The basic workflow of GitHub is:
Create a new repository for your code on GitHub
Add this as a remote for your local repository
Make changes to your local repository as usual
Upload or push your changes to the remote
*For Continuing Further We recommend you to get started with GitHub Account Sign Up and all. Hope that if you are learning Git&GitHub then you would be able to create an account on your own. In case of any obstacle, you can comment below for help.
Creating a repository on GitHub
You need to find this Section on your GitHub Profile.
Click on Create Repository button.
Now Fill in the Details as per your requirements. The Basic Demo is shown as
Give your repository a useful name and description. You'll need to leave it as a public repository (private repositories are currently only available with a paid-for plan). For this web thread, leave the option to initialize the repository with a README unticked, and don't select a .gitignore file or license. Then click the big green "Create Repository" button.
The final stage provides you with the command line instructions you need for adding the GitHub repository as a remote for your local copy.
Note the option to switch between ssh and https, and how this changes the address for the repository. Stick with https for now
We'll add this repository as a remote for the repository we created earlier, so follow the instructions in the second box, titled "... or push an existing repository from the command line".
In our repository folder that we created earlier, type the following commands, substituting your GitHub username and the repository name into the appropriate places:
git remote add origin https://github.com/<yourusename>/<yourreponame>.git git push -u origin master
The term "master" refers to the default or master branch in the repository. Branches are effectively self-contained copies of the files in the project that can be merged when required. They are often used for the development and stable versions of source code.
Go back to your local repository and practice making some more changes to your local repository, then use git push to push them to the remote repository. Now you might see the value of good commit messages, as these are fully visible in your repository!
Note that once you have set the master branch you don't need to use the full "-u origin master" statement. Just "git push" will do.
Next We shall look after GitHub Basics and Setup by next Weekend In this Thread itself. Hope Our Web Guide to Git helped you to learn the fundamentals.